R Warrior is here
An R programming game based on Ruby Warrior
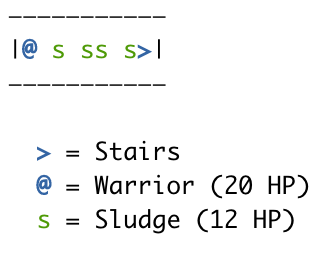
About a decade ago, I discovered the game Ruby Warrior by Ryan Bates. The concept was simple: get to the top of the tower to reach the precious Ruby. Instead of controlling the warrior directly, you write an AI to conquer each level. Levels start off very basic, with the first level simply requiring you to walk straight to the stairs (the end of the level), while the second level introduces an enemy to defeat, and the third a horde of enemies that requires resting to restore health. This is a game that can help you learn a programming language!
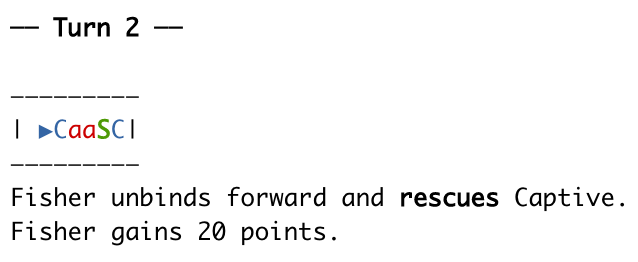
Level 5 beginner screenshot
Ruby Warrior implemented two towers (level sets): the beginner being a one-dimensional hall, while the intermediate tower extends the game into two dimensions. Once you get through each level of a tower, an epic mode becomes available where a single AI must get to the top of the tower. You can then refine your AI so that it achieves an S grade for each level.
My only problem was I didn’t know Ruby well, so I was a bit slow to get through the game, though that was kind of the point, to learn the language while playing the game. That didn’t stop me from wanting to implement the game in R.
As far as I’m aware, there’s not another programming game in existence implemented in R. At least it’s not on this list of R games by Matt Dray.
Installation
Install from CRAN:
install.packages("rwarrior")
Or the development version from Github:
# install.packages("devtools") # If devtools is not installed
devtools::install_github("trickytank/rwarrior", build_vignettes = TRUE)
Gameplay
The goal of R warrior is to reach the top of the tower and find the precious Hex.
You should start playing from level 1 to write your first AI.
The level_readme()
function gives the commands available to your warrior.
Read this first before attempting to write your AI for each level.
library(rwarrior)
level_readme(1)
## Level 1
## You see before yourself a long hallway with stairs at the end. There is nothing in the way.
##
## Tip: Call warrior$walk() to walk forward in your AI.
##
## ——————————
## |▶ ▟|
## ——————————
##
## ▟ = Stairs
## ▶ = Warrior (20 HP)
##
## - warrior$walk(direction = "forward")
## Move the warrior in the given direction, any of:
## - "forward"
## - "backward"
##
## ── Warrior actions (can only call one per turn):
From this, write your AI function, which has two arguments. A template AI function is given below:
# Modify the AI function
AI <- function(warrior, memory) {
if(is.null(memory)) {
# give initial values when memory is NULL
memory <- list(variable1 = "initial value")
}
# Your code goes here #
# Access memory variable1 with memory$variable1
# The AI result should be the memory,
# this is passed onto the next call of your AI.
return(memory)
}
warrior_name <- "Fisher" # A name for your warrior
play_warrior(AI, warrior_name = warrior_name, level = 1)
Hopefully, you passed the first level.
Let me know in the comments if these instructions or level_readme()
are unclear.
Once you complete the nine beginner levels, it’s time to try epic mode.
play_epic(AI, warrior_name = warrior_name)
You receive a grade for each level.
When improving your AI, you may not want to attempt all levels at once.
Use practice = TRUE
in play_warrior()
to allow your AI to use all abilities available in the top level of that tower.
play_warrior(AI, warrior_name = warrior_name, level = 1, practice = TRUE)
Let me know your best overall grades and grade percentage, but please don’t post your best AIs (though you can email them to me at rickmtankard@gmail.com).
Future directions
- Submit to CRAN (with beginner towers initially).
- Add intermediate towers (9 more levels).
- Create a graphical representation of the warrior’s movement. Hit me up if this is something you would be interested in helping out in, either on the programming side or creating the sprites. I’d like to see the warrior in Pixel Art, but there would be room to have more than one skin available.
- Create a Hex logo with a pixel-art warrior (as used in the graphical representation).
- Write my own AI to complete the epic mode beginner tower with S rank on all levels to find if it’s possible, and adjust the ace score (target score for an S rank) accordingly.
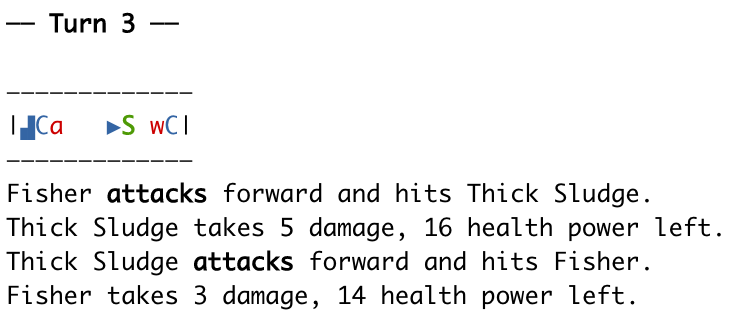
Level 9 beginner screenshot
Final words
I’d love to hear about anybody’s experience playing R warrior. There are no doubt some bugs (as of writing, I know of one) and some unclear documentation that I’d like feedback on.
I plan to post more about R Warrior’s development journey and a few things I’ve learnt on the way.